mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-10-18 22:30:39 +00:00
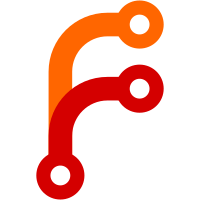
* imlemented `CameraSpotDetector` * separated generic `DialogSwitch` * added `CameraFeature` model * added `CameraFeaturesListTile` to metering section * added features dialog subtitles * added long press to remove metering spot * translations * hide camera features for purchasable status * hide `CameraHistogram` & `CameraSpotDetector` if purchasable * bumped iap version * fixed tests * removed redundant camera state emission * tests * Fixed remote config initalization * updated pro features description
65 lines
1.8 KiB
Dart
65 lines
1.8 KiB
Dart
// ignore_for_file: prefer_const_constructors
|
|
|
|
import 'dart:ui';
|
|
|
|
import 'package:lightmeter/screens/metering/components/camera_container/event_container_camera.dart';
|
|
import 'package:test/test.dart';
|
|
|
|
void main() {
|
|
group(
|
|
'`ZoomChangedEvent`',
|
|
() {
|
|
final a = ZoomChangedEvent(1.0);
|
|
final b = ZoomChangedEvent(1.0);
|
|
final c = ZoomChangedEvent(2.0);
|
|
test('==', () {
|
|
expect(a == b && b == a, true);
|
|
expect(a != c && c != a, true);
|
|
expect(b != c && c != b, true);
|
|
});
|
|
test('hashCode', () {
|
|
expect(a.hashCode == b.hashCode, true);
|
|
expect(a.hashCode != c.hashCode, true);
|
|
expect(b.hashCode != c.hashCode, true);
|
|
});
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`ExposureOffsetChangedEvent`',
|
|
() {
|
|
final a = ExposureOffsetChangedEvent(1.0);
|
|
final b = ExposureOffsetChangedEvent(1.0);
|
|
final c = ExposureOffsetChangedEvent(2.0);
|
|
test('==', () {
|
|
expect(a == b && b == a, true);
|
|
expect(a != c && c != a, true);
|
|
expect(b != c && c != b, true);
|
|
});
|
|
test('hashCode', () {
|
|
expect(a.hashCode == b.hashCode, true);
|
|
expect(a.hashCode != c.hashCode, true);
|
|
expect(b.hashCode != c.hashCode, true);
|
|
});
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`ExposureSpotChangedEvent`',
|
|
() {
|
|
final a = ExposureSpotChangedEvent(Offset(0.0, 0.0));
|
|
final b = ExposureSpotChangedEvent(Offset(0.0, 0.0));
|
|
final c = ExposureSpotChangedEvent(Offset(2.0, 2.0));
|
|
test('==', () {
|
|
expect(a == b && b == a, true);
|
|
expect(a != c && c != a, true);
|
|
expect(b != c && c != b, true);
|
|
});
|
|
test('hashCode', () {
|
|
expect(a.hashCode == b.hashCode, true);
|
|
expect(a.hashCode != c.hashCode, true);
|
|
expect(b.hashCode != c.hashCode, true);
|
|
});
|
|
},
|
|
);
|
|
}
|