mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-11-21 23:10:40 +00:00
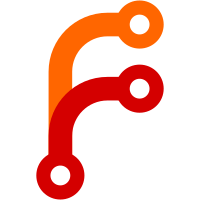
- Fixed test coverage calculation - Removed `mockito` from the application mock - Implemented platform channel mocks to mimic incident light metering - Covered providers with unit tests - Covered metering screen pickers with widget tests - Laid foundation for integration tests
29 lines
779 B
Dart
29 lines
779 B
Dart
import 'package:flutter/material.dart';
|
|
|
|
enum SelectableAspect { list, selected }
|
|
|
|
class SelectableInheritedModel<T> extends InheritedModel<SelectableAspect> {
|
|
const SelectableInheritedModel({
|
|
super.key,
|
|
required this.values,
|
|
required this.selected,
|
|
required super.child,
|
|
});
|
|
|
|
final List<T> values;
|
|
final T selected;
|
|
|
|
@override
|
|
bool updateShouldNotify(SelectableInheritedModel oldWidget) => true;
|
|
|
|
@override
|
|
bool updateShouldNotifyDependent(SelectableInheritedModel oldWidget, Set<SelectableAspect> dependencies) {
|
|
if (dependencies.contains(SelectableAspect.list)) {
|
|
return true;
|
|
} else if (dependencies.contains(SelectableAspect.selected)) {
|
|
return selected != oldWidget.selected;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
}
|