mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-11-22 07:20:39 +00:00
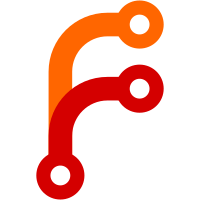
* integrated `EquipmentProfilesStorageService` * implemented `EquipmentProfileEditScreen` * added equipment profiles screens to navigation * fixed tests * fixed splashscreen removal * replaced old `EquipmentProfilesScreen` * typo * use outlined icons * fixed storage mock for integration tests * recovered copy feature for profiles * added profile deletion to e2e test * added translations * added film translations * wip * add ability to toggle equipment profiles * lints * fixed tests * sync with iap rename * use `Toggleable` from resources * use iap 2.1.0 * use outlined edit icon
118 lines
5 KiB
Dart
118 lines
5 KiB
Dart
import 'package:flutter/material.dart';
|
|
import 'package:flutter_bloc/flutter_bloc.dart';
|
|
import 'package:lightmeter/generated/l10n.dart';
|
|
import 'package:lightmeter/res/dimens.dart';
|
|
import 'package:lightmeter/screens/film_edit/bloc_film_edit.dart';
|
|
import 'package:lightmeter/screens/film_edit/components/exponential_formula_input/widget_input_exponential_formula_film_edit.dart';
|
|
import 'package:lightmeter/screens/film_edit/components/iso_picker/widget_picker_iso_film_edit.dart';
|
|
import 'package:lightmeter/screens/film_edit/components/name_field/widget_field_name_film_edit.dart';
|
|
import 'package:lightmeter/screens/film_edit/event_film_edit.dart';
|
|
import 'package:lightmeter/screens/film_edit/state_film_edit.dart';
|
|
import 'package:lightmeter/screens/shared/sliver_screen/screen_sliver.dart';
|
|
|
|
class FilmEditScreen extends StatefulWidget {
|
|
final bool isEdit;
|
|
|
|
const FilmEditScreen({
|
|
required this.isEdit,
|
|
super.key,
|
|
});
|
|
|
|
@override
|
|
State<FilmEditScreen> createState() => _FilmEditScreenState();
|
|
}
|
|
|
|
class _FilmEditScreenState extends State<FilmEditScreen> {
|
|
@override
|
|
Widget build(BuildContext context) {
|
|
return BlocConsumer<FilmEditBloc, FilmEditState>(
|
|
listenWhen: (previous, current) => previous.isLoading != current.isLoading,
|
|
listener: (context, state) {
|
|
if (state.isLoading) {
|
|
FocusScope.of(context).unfocus();
|
|
} else {
|
|
Navigator.of(context).pop();
|
|
}
|
|
},
|
|
buildWhen: (previous, current) => previous.isLoading != current.isLoading,
|
|
builder: (context, state) => IgnorePointer(
|
|
ignoring: state.isLoading,
|
|
child: SliverScreen(
|
|
title: Text(widget.isEdit ? S.of(context).editFilmTitle : S.of(context).addFilmTitle),
|
|
appBarActions: [
|
|
BlocBuilder<FilmEditBloc, FilmEditState>(
|
|
buildWhen: (previous, current) => previous.canSave != current.canSave,
|
|
builder: (context, state) => IconButton(
|
|
onPressed: state.canSave
|
|
? () {
|
|
context.read<FilmEditBloc>().add(const FilmEditSaveEvent());
|
|
}
|
|
: null,
|
|
icon: const Icon(Icons.save_outlined),
|
|
),
|
|
),
|
|
if (widget.isEdit)
|
|
IconButton(
|
|
onPressed: () {
|
|
context.read<FilmEditBloc>().add(const FilmEditDeleteEvent());
|
|
},
|
|
icon: const Icon(Icons.delete_outlined),
|
|
),
|
|
],
|
|
slivers: [
|
|
SliverToBoxAdapter(
|
|
child: Padding(
|
|
padding: const EdgeInsets.fromLTRB(
|
|
Dimens.paddingM,
|
|
0,
|
|
Dimens.paddingM,
|
|
Dimens.paddingM,
|
|
),
|
|
child: Card(
|
|
child: Padding(
|
|
padding: const EdgeInsets.symmetric(vertical: Dimens.paddingM),
|
|
child: Opacity(
|
|
opacity: state.isLoading ? Dimens.disabledOpacity : Dimens.enabledOpacity,
|
|
child: Column(
|
|
children: [
|
|
BlocBuilder<FilmEditBloc, FilmEditState>(
|
|
buildWhen: (previous, current) => previous.name != current.name,
|
|
builder: (context, state) => FilmEditNameField(
|
|
name: state.name,
|
|
onChanged: (value) {
|
|
context.read<FilmEditBloc>().add(FilmEditNameChangedEvent(value));
|
|
},
|
|
),
|
|
),
|
|
BlocBuilder<FilmEditBloc, FilmEditState>(
|
|
buildWhen: (previous, current) => previous.isoValue != current.isoValue,
|
|
builder: (context, state) => FilmEditIsoPicker(
|
|
selected: state.isoValue,
|
|
onChanged: (value) {
|
|
context.read<FilmEditBloc>().add(FilmEditIsoChangedEvent(value));
|
|
},
|
|
),
|
|
),
|
|
BlocBuilder<FilmEditBloc, FilmEditState>(
|
|
buildWhen: (previous, current) => previous.exponent != current.exponent,
|
|
builder: (context, state) => FilmEditExponentialFormulaInput(
|
|
value: state.exponent,
|
|
onChanged: (value) {
|
|
context.read<FilmEditBloc>().add(FilmEditExpChangedEvent(value));
|
|
},
|
|
),
|
|
),
|
|
],
|
|
),
|
|
),
|
|
),
|
|
),
|
|
),
|
|
),
|
|
SliverToBoxAdapter(child: SizedBox(height: MediaQuery.paddingOf(context).bottom)),
|
|
],
|
|
),
|
|
),
|
|
);
|
|
}
|
|
}
|