mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-10-18 14:20:40 +00:00
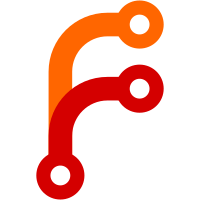
* removed redundant `UserPreferencesService` from `MeteringBloc` * wip * post-merge fixes * `MeasureEvent` tests * `MeasureEvent` tests revision * `MeasureEvent` tests added timeout * added stubs for other `MeteringBloc` events * rewritten `MeteringBloc` logic * wip * `IsoChangedEvent` tests * refined `IsoChangedEvent` tests * `NdChangedEvent` tests * `FilmChangedEvent` tests * `MeteringCommunicationBloc` tests * added test run to ci * overriden `==` for `MeasuredState` * `LuxMeteringEvent` tests * refined `LuxMeteringEvent` tests * rename * wip * wip * `InitializeEvent`/`DeinitializeEvent` tests * clamp minZoomLevel * fixed `MeteringCommunicationBloc` tests * wip * `ZoomChangedEvent` tests * `ExposureOffsetChangedEvent`/`ExposureOffsetResetEvent` tests * renamed test groups * added test coverage script * improved `CameraContainerBloc` test coverage * `EquipmentProfileChangedEvent` tests * verify response vibration * fixed running all tests * `MeteringCommunicationBloc` equality tests * `CameraContainerBloc` equality tests * removed generated code from coverage * `MeteringScreenLayoutFeature` tests * `SupportedLocale` tests * `Film` tests * `CaffeineService` tests * `UserPreferencesService` tests (wip) * `LightSensorService` tests (wip) * `migrateOldKeys()` tests * ignore currently unused getters & setters * gradle upgrade * `reset(sharedPreferences);` calls count * typo * `MeteringInteractor` tests * `SettingsInteractor` tests (wip) * `MeteringInteractor` tests (wip) * `SettingsInteractor` tests
63 lines
2.3 KiB
Dart
63 lines
2.3 KiB
Dart
import 'package:lightmeter/data/caffeine_service.dart';
|
|
import 'package:lightmeter/data/haptics_service.dart';
|
|
import 'package:lightmeter/data/models/volume_action.dart';
|
|
import 'package:lightmeter/data/shared_prefs_service.dart';
|
|
import 'package:lightmeter/data/volume_events_service.dart';
|
|
|
|
class SettingsInteractor {
|
|
final UserPreferencesService _userPreferencesService;
|
|
final CaffeineService _caffeineService;
|
|
final HapticsService _hapticsService;
|
|
final VolumeEventsService _volumeEventsService;
|
|
|
|
const SettingsInteractor(
|
|
this._userPreferencesService,
|
|
this._caffeineService,
|
|
this._hapticsService,
|
|
this._volumeEventsService,
|
|
);
|
|
|
|
double get cameraEvCalibration => _userPreferencesService.cameraEvCalibration;
|
|
void setCameraEvCalibration(double value) => _userPreferencesService.cameraEvCalibration = value;
|
|
|
|
double get lightSensorEvCalibration => _userPreferencesService.lightSensorEvCalibration;
|
|
void setLightSensorEvCalibration(double value) =>
|
|
_userPreferencesService.lightSensorEvCalibration = value;
|
|
|
|
bool get isCaffeineEnabled => _userPreferencesService.caffeine;
|
|
Future<void> enableCaffeine(bool enable) async {
|
|
await _caffeineService.keepScreenOn(enable).then((value) {
|
|
_userPreferencesService.caffeine = enable;
|
|
});
|
|
}
|
|
|
|
Future<void> disableVolumeHandling() async {
|
|
await _volumeEventsService.setVolumeHandling(false);
|
|
}
|
|
Future<void> restoreVolumeHandling() async {
|
|
await _volumeEventsService
|
|
.setVolumeHandling(_userPreferencesService.volumeAction != VolumeAction.none);
|
|
}
|
|
|
|
VolumeAction get volumeAction => _userPreferencesService.volumeAction;
|
|
Future<void> setVolumeAction(VolumeAction value) async {
|
|
_userPreferencesService.volumeAction = value;
|
|
await _volumeEventsService.setVolumeHandling(value != VolumeAction.none);
|
|
}
|
|
|
|
bool get isHapticsEnabled => _userPreferencesService.haptics;
|
|
void enableHaptics(bool enable) {
|
|
_userPreferencesService.haptics = enable;
|
|
quickVibration();
|
|
}
|
|
|
|
/// Executes vibration if haptics are enabled in settings
|
|
void quickVibration() {
|
|
if (_userPreferencesService.haptics) _hapticsService.quickVibration();
|
|
}
|
|
|
|
/// Executes vibration if haptics are enabled in settings
|
|
void responseVibration() {
|
|
if (_userPreferencesService.haptics) _hapticsService.responseVibration();
|
|
}
|
|
}
|