mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-10-18 14:20:40 +00:00
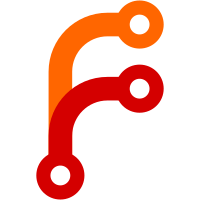
* [Android] wip * implemented `VolumeEventsService` * implemented `VolumeKeysListener` (wip) * Added screenshots links * [Android] nullable typo * implemented `VolumeKeysNotifier` * deinitialize camera when on Settings screen * disable volume handling when on Settings screen * used "platform" package to mock `isAndroid` * init/deinit camera on settings open * allow volume action override only on metering screen * lints * cleanup * await dispose * tests * reduced `SwitchListTile.contentPadding` * fixed tests * removed `VolumeAction.zoom` * added social preview * typo * fixed `CameraContainerBloc` tests * added `Stream.empty()` tests
63 lines
2.3 KiB
Dart
63 lines
2.3 KiB
Dart
import 'package:lightmeter/data/caffeine_service.dart';
|
|
import 'package:lightmeter/data/haptics_service.dart';
|
|
import 'package:lightmeter/data/models/volume_action.dart';
|
|
import 'package:lightmeter/data/shared_prefs_service.dart';
|
|
import 'package:lightmeter/data/volume_events_service.dart';
|
|
|
|
class SettingsInteractor {
|
|
final UserPreferencesService _userPreferencesService;
|
|
final CaffeineService _caffeineService;
|
|
final HapticsService _hapticsService;
|
|
final VolumeEventsService _volumeEventsService;
|
|
|
|
const SettingsInteractor(
|
|
this._userPreferencesService,
|
|
this._caffeineService,
|
|
this._hapticsService,
|
|
this._volumeEventsService,
|
|
);
|
|
|
|
double get cameraEvCalibration => _userPreferencesService.cameraEvCalibration;
|
|
void setCameraEvCalibration(double value) => _userPreferencesService.cameraEvCalibration = value;
|
|
|
|
double get lightSensorEvCalibration => _userPreferencesService.lightSensorEvCalibration;
|
|
void setLightSensorEvCalibration(double value) =>
|
|
_userPreferencesService.lightSensorEvCalibration = value;
|
|
|
|
bool get isCaffeineEnabled => _userPreferencesService.caffeine;
|
|
Future<void> enableCaffeine(bool enable) async {
|
|
await _caffeineService.keepScreenOn(enable).then((value) {
|
|
_userPreferencesService.caffeine = enable;
|
|
});
|
|
}
|
|
|
|
Future<void> disableVolumeHandling() async {
|
|
await _volumeEventsService.setVolumeHandling(false);
|
|
}
|
|
Future<void> restoreVolumeHandling() async {
|
|
await _volumeEventsService
|
|
.setVolumeHandling(_userPreferencesService.volumeAction != VolumeAction.none);
|
|
}
|
|
|
|
VolumeAction get volumeAction => _userPreferencesService.volumeAction;
|
|
Future<void> setVolumeAction(VolumeAction value) async {
|
|
await _volumeEventsService.setVolumeHandling(value != VolumeAction.none);
|
|
_userPreferencesService.volumeAction = value;
|
|
}
|
|
|
|
bool get isHapticsEnabled => _userPreferencesService.haptics;
|
|
void enableHaptics(bool enable) {
|
|
_userPreferencesService.haptics = enable;
|
|
quickVibration();
|
|
}
|
|
|
|
/// Executes vibration if haptics are enabled in settings
|
|
void quickVibration() {
|
|
if (_userPreferencesService.haptics) _hapticsService.quickVibration();
|
|
}
|
|
|
|
/// Executes vibration if haptics are enabled in settings
|
|
void responseVibration() {
|
|
if (_userPreferencesService.haptics) _hapticsService.responseVibration();
|
|
}
|
|
}
|