mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-11-22 23:40:41 +00:00
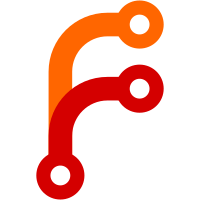
* removed redundant `UserPreferencesService` from `MeteringBloc` * wip * post-merge fixes * `MeasureEvent` tests * `MeasureEvent` tests revision * `MeasureEvent` tests added timeout * added stubs for other `MeteringBloc` events * rewritten `MeteringBloc` logic * wip * `IsoChangedEvent` tests * refined `IsoChangedEvent` tests * `NdChangedEvent` tests * `FilmChangedEvent` tests * `MeteringCommunicationBloc` tests * added test run to ci * overriden `==` for `MeasuredState` * `LuxMeteringEvent` tests * refined `LuxMeteringEvent` tests * rename * wip * wip * `InitializeEvent`/`DeinitializeEvent` tests * clamp minZoomLevel * fixed `MeteringCommunicationBloc` tests * wip * `ZoomChangedEvent` tests * `ExposureOffsetChangedEvent`/`ExposureOffsetResetEvent` tests * renamed test groups * added test coverage script * improved `CameraContainerBloc` test coverage * `EquipmentProfileChangedEvent` tests * verify response vibration * fixed running all tests * `MeteringCommunicationBloc` equality tests * `CameraContainerBloc` equality tests * removed generated code from coverage
44 lines
1.2 KiB
Dart
44 lines
1.2 KiB
Dart
// ignore_for_file: prefer_const_constructors
|
|
|
|
import 'package:lightmeter/screens/metering/communication/event_communication_metering.dart';
|
|
import 'package:test/test.dart';
|
|
|
|
void main() {
|
|
group(
|
|
'`MeteringInProgressEvent`',
|
|
() {
|
|
final a = MeteringInProgressEvent(1.0);
|
|
final b = MeteringInProgressEvent(1.0);
|
|
final c = MeteringInProgressEvent(2.0);
|
|
test('==', () {
|
|
expect(a == b && b == a, true);
|
|
expect(a != c && c != a, true);
|
|
expect(b != c && c != b, true);
|
|
});
|
|
test('hashCode', () {
|
|
expect(a.hashCode == b.hashCode, true);
|
|
expect(a.hashCode != c.hashCode, true);
|
|
expect(b.hashCode != c.hashCode, true);
|
|
});
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`MeteringEndedEvent`',
|
|
() {
|
|
final a = MeteringEndedEvent(1.0);
|
|
final b = MeteringEndedEvent(1.0);
|
|
final c = MeteringEndedEvent(2.0);
|
|
test('==', () {
|
|
expect(a == b && b == a, true);
|
|
expect(a != c && c != a, true);
|
|
expect(b != c && c != b, true);
|
|
});
|
|
test('hashCode', () {
|
|
expect(a.hashCode == b.hashCode, true);
|
|
expect(a.hashCode != c.hashCode, true);
|
|
expect(b.hashCode != c.hashCode, true);
|
|
});
|
|
},
|
|
);
|
|
}
|