mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-11-22 07:20:39 +00:00
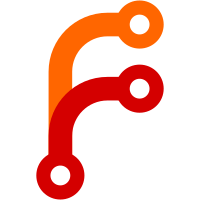
* wip * added start/stop button * animated timeline * fixed timer stop state * added reset button (wip) * added `onExposurePairTap` callback * integrated `TimerScreen` to navigation * separated `TimerTimeline` * fixed timeline flickering * added milliseconds to timer * synchronized timeline with actual timer * reused `BottomControlsBar` * fixed default scaffold background color * moved center button size to the bar itself * display selected exposure pair on timer screen * separated reusable `AnimatedCircluarButton` * release camera when timer is opened * added `TimerInteractor` * added `TimerBloc` test * fixed hours parsing * added scenarios for timer golden test * adjusted timer timeline colors * show iso & nd values on timer screen * automatically close timer screen after timeout * added timer autostart * reverted theme changes * updated goldens * typo * removed timer screen auto-dismiss * increased timer vibration duration * replaced outlined locks * increased 1/3 values font size
41 lines
1.3 KiB
Dart
41 lines
1.3 KiB
Dart
import 'dart:async';
|
|
|
|
import 'package:flutter_bloc/flutter_bloc.dart';
|
|
import 'package:lightmeter/interactors/timer_interactor.dart';
|
|
import 'package:lightmeter/screens/timer/event_timer.dart';
|
|
import 'package:lightmeter/screens/timer/state_timer.dart';
|
|
|
|
class TimerBloc extends Bloc<TimerEvent, TimerState> {
|
|
final TimerInteractor _timerInteractor;
|
|
final Duration duration;
|
|
|
|
TimerBloc(this._timerInteractor, this.duration) : super(const TimerStoppedState()) {
|
|
on<StartTimerEvent>(_onStartTimer);
|
|
on<TimerEndedEvent>(_onTimerEnded);
|
|
on<StopTimerEvent>(_onStopTimer);
|
|
on<ResetTimerEvent>(_onResetTimer);
|
|
|
|
if (_timerInteractor.isAutostartTimerEnabled) add(const StartTimerEvent());
|
|
}
|
|
|
|
Future<void> _onStartTimer(StartTimerEvent _, Emitter emit) async {
|
|
_timerInteractor.startVibration();
|
|
emit(const TimerResumedState());
|
|
}
|
|
|
|
Future<void> _onTimerEnded(TimerEndedEvent event, Emitter emit) async {
|
|
if (state is! TimerResetState) {
|
|
_timerInteractor.endVibration();
|
|
emit(const TimerStoppedState());
|
|
}
|
|
}
|
|
|
|
Future<void> _onStopTimer(StopTimerEvent _, Emitter emit) async {
|
|
_timerInteractor.startVibration();
|
|
emit(const TimerStoppedState());
|
|
}
|
|
|
|
Future<void> _onResetTimer(ResetTimerEvent _, Emitter emit) async {
|
|
emit(const TimerResetState());
|
|
}
|
|
}
|