mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-11-22 15:30:59 +00:00
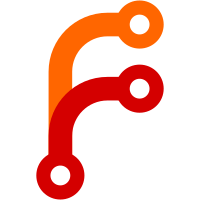
* test granting and revoking pro features * extracted common widget tester actions * test disabling & enabling of the metering screen layout features * added integration tests to CI * added integration tests to PR check * allow matrix jobs to fail * use base64 -d * downgraded iphone version to the supported one * use proper android device name * typo in macos version * upgraded iphone version to the supported one * updated android compileSdkVersion * added google services json restoration * combined all tests in one file * removed ipa signing for ios test * debug prints :) * lints * refined tester extension and expectations * e2e test (wip) * added more expectations to e2e test * changed pickers order a bit in e2e test * added equipment profiles creation to e2e test * added film selection to e2e test * set android emulator API level to 32 * use flutter drive for integration tests * removed app pre-build * try running tests only for one platform * added no-dds to flutter drive * try running only on ios * bumped macos version * increased tests timeout * set IPHONEOS_DEPLOYMENT_TARGET = 12.0 * removed prints * Update Podfile * restore firebase_app_id_file.json * Delete run_integration_tests.sh * run e2e with all tests * reverted pr-check
35 lines
1.7 KiB
Dart
35 lines
1.7 KiB
Dart
import 'package:flutter/widgets.dart';
|
|
import 'package:flutter_test/flutter_test.dart';
|
|
import 'package:lightmeter/screens/metering/components/shared/exposure_pairs_list/components/exposure_pairs_list_item/widget_item_list_exposure_pairs.dart';
|
|
import 'package:lightmeter/screens/metering/components/shared/exposure_pairs_list/widget_list_exposure_pairs.dart';
|
|
import 'package:lightmeter/screens/metering/components/shared/readings_container/components/extreme_exposure_pairs_container/widget_container_extreme_exposure_pairs.dart';
|
|
import 'package:m3_lightmeter_resources/m3_lightmeter_resources.dart';
|
|
|
|
void expectPickerTitle<P extends Widget>(String title, {String? reason}) {
|
|
expect(find.descendant(of: find.byType(P), matching: find.text(title)), findsOneWidget, reason: reason);
|
|
}
|
|
|
|
void expectExtremeExposurePairs(String fastest, String slowest, {String? reason}) {
|
|
final pickerFinder = find.byType(ExtremeExposurePairsContainer);
|
|
expect(find.descendant(of: pickerFinder, matching: find.text(fastest)), findsOneWidget, reason: reason);
|
|
expect(find.descendant(of: pickerFinder, matching: find.text(slowest)), findsOneWidget, reason: reason);
|
|
}
|
|
|
|
void expectExposurePairsListItem(WidgetTester tester, String aperture, String shutterSpeed, {String? reason}) {
|
|
Key? findKey<T extends PhotographyStopValue<num>>(String value) => tester
|
|
.widget<Row>(
|
|
find.ancestor(
|
|
of: find.ancestor(
|
|
of: find.text(value),
|
|
matching: find.byType(ExposurePairsListItem<T>),
|
|
),
|
|
matching: find.descendant(of: find.byType(ExposurePairsList), matching: find.byType(Row)),
|
|
),
|
|
)
|
|
.key;
|
|
expect(
|
|
findKey<ApertureValue>(aperture),
|
|
findKey<ShutterSpeedValue>(shutterSpeed),
|
|
reason: reason,
|
|
);
|
|
}
|