mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-10-18 22:30:39 +00:00
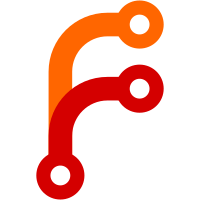
* removed redundant `UserPreferencesService` from `MeteringBloc` * wip * post-merge fixes * `MeasureEvent` tests * `MeasureEvent` tests revision * `MeasureEvent` tests added timeout * added stubs for other `MeteringBloc` events * rewritten `MeteringBloc` logic * wip * `IsoChangedEvent` tests * refined `IsoChangedEvent` tests * `NdChangedEvent` tests * `FilmChangedEvent` tests * `MeteringCommunicationBloc` tests * added test run to ci * overriden `==` for `MeasuredState` * `LuxMeteringEvent` tests * refined `LuxMeteringEvent` tests * rename * wip * wip * `InitializeEvent`/`DeinitializeEvent` tests * clamp minZoomLevel * fixed `MeteringCommunicationBloc` tests * wip * `ZoomChangedEvent` tests * `ExposureOffsetChangedEvent`/`ExposureOffsetResetEvent` tests * renamed test groups * added test coverage script * improved `CameraContainerBloc` test coverage * `EquipmentProfileChangedEvent` tests * verify response vibration * fixed running all tests * `MeteringCommunicationBloc` equality tests * `CameraContainerBloc` equality tests * removed generated code from coverage * `MeteringScreenLayoutFeature` tests * `SupportedLocale` tests * `Film` tests * `CaffeineService` tests * `UserPreferencesService` tests (wip) * `LightSensorService` tests (wip) * `migrateOldKeys()` tests * ignore currently unused getters & setters * gradle upgrade * `reset(sharedPreferences);` calls count * typo * `MeteringInteractor` tests * `SettingsInteractor` tests (wip) * `MeteringInteractor` tests (wip) * `SettingsInteractor` tests * AnimatedDialog picker standalone tests * Moved Animated dialog picker to widget tests * `ExtremeExposurePairsContainer` widget test * dialog picker test * Match extreme exposure pairs & pairs list edge values * `FilmPicker` widget tests * fixed animated dialog picker tests * add not hit files to coverage percentage * Moved `EquipmentProfileProvider` & `FilmsProvider` to the main repo * Synced _iap_ stub with repo * `FilmsProvider` tests * `EquipmentProfileProvider` tests * Pass `availableFilms` to `FilmsProvider` * `FilmPicker` tests * removed unnecessary imports * Metering layout features tests * split integration tests by screens * Films in use test * mock light meter lux stream * removed mockito mocks for integration tests From no on these are the only mocks in use: - Mock shared prefs initial values - Mock platform responses (camera/light sensor) * set sharedprefs mock without redundant group * unified granting camera permission on Android * fixed metering screen tests * extracted common values * `FilmPicker` integration tests * fixed light sensor platform mocks * wip * removed integration tests for now * moved screenshots generator to screenshots folder * typo * removed `MockIAPProductsProvider` * implemented platform mocks for unit tests * data/models/ 100% coverage * `IsoValuePicker` tests * `EquipmentProfileProvider` tests * extended PR check timeout * typo * added storage action verification for `FilmsProvider` tests * `UserPreferencesProvider` tests * Update README.md * added //coverage:ignore to `ServicesProvider` * typo * typo * `toStringSignedAsFixed` tests * `SelectableInheritedModel` tests * removed unused `TextLineHeight` util * `VolumeKeysNotifier` tests * import * `EquipmentProfileListener` tests * typo * split `EquipmentProfileListener` tests * `showBuyProDialog` tests * added `maybeOf` getter for iap stub
537 lines
20 KiB
Dart
537 lines
20 KiB
Dart
import 'package:bloc_test/bloc_test.dart';
|
|
import 'package:lightmeter/data/models/volume_action.dart';
|
|
import 'package:lightmeter/interactors/metering_interactor.dart';
|
|
import 'package:lightmeter/screens/metering/bloc_metering.dart';
|
|
import 'package:lightmeter/screens/metering/communication/bloc_communication_metering.dart';
|
|
import 'package:lightmeter/screens/metering/communication/event_communication_metering.dart'
|
|
as communication_events;
|
|
import 'package:lightmeter/screens/metering/communication/state_communication_metering.dart'
|
|
as communication_states;
|
|
import 'package:lightmeter/screens/metering/event_metering.dart';
|
|
import 'package:lightmeter/screens/metering/state_metering.dart';
|
|
import 'package:lightmeter/screens/metering/utils/notifier_volume_keys.dart';
|
|
import 'package:m3_lightmeter_resources/m3_lightmeter_resources.dart';
|
|
import 'package:mocktail/mocktail.dart';
|
|
import 'package:test/test.dart';
|
|
|
|
class _MockMeteringInteractor extends Mock implements MeteringInteractor {}
|
|
|
|
class _MockVolumeKeysNotifier extends Mock implements VolumeKeysNotifier {}
|
|
|
|
class _MockMeteringCommunicationBloc extends MockBloc<
|
|
communication_events.MeteringCommunicationEvent,
|
|
communication_states.MeteringCommunicationState> implements MeteringCommunicationBloc {}
|
|
|
|
void main() {
|
|
late _MockMeteringInteractor meteringInteractor;
|
|
late _MockVolumeKeysNotifier volumeKeysNotifier;
|
|
late _MockMeteringCommunicationBloc communicationBloc;
|
|
late MeteringBloc bloc;
|
|
const iso100 = IsoValue(100, StopType.full);
|
|
|
|
setUp(() {
|
|
meteringInteractor = _MockMeteringInteractor();
|
|
when<IsoValue>(() => meteringInteractor.iso).thenReturn(iso100);
|
|
when<NdValue>(() => meteringInteractor.ndFilter).thenReturn(NdValue.values.first);
|
|
when(meteringInteractor.quickVibration).thenAnswer((_) async {});
|
|
when(meteringInteractor.responseVibration).thenAnswer((_) async {});
|
|
when(meteringInteractor.errorVibration).thenAnswer((_) async {});
|
|
|
|
volumeKeysNotifier = _MockVolumeKeysNotifier();
|
|
communicationBloc = _MockMeteringCommunicationBloc();
|
|
|
|
bloc = MeteringBloc(
|
|
meteringInteractor,
|
|
volumeKeysNotifier,
|
|
communicationBloc,
|
|
);
|
|
});
|
|
|
|
tearDown(() {
|
|
bloc.close();
|
|
//volumeKeysNotifier.dispose();
|
|
communicationBloc.close();
|
|
});
|
|
|
|
group(
|
|
'`MeasureEvent`',
|
|
() {
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'`MeasureEvent` -> success',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.add(const MeasureEvent());
|
|
bloc.onCommunicationState(const communication_states.MeteringEndedState(2));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.quickVibration()).called(1);
|
|
verify(() => communicationBloc.add(const communication_events.MeasureEvent())).called(1);
|
|
verify(() => meteringInteractor.responseVibration()).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<LoadingState>(),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.isMetering, 'isMetering', false)
|
|
.having((state) => state.ev, 'ev', 2),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'`MeasureEvent` -> error',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.add(const MeasureEvent());
|
|
bloc.onCommunicationState(const communication_states.MeteringEndedState(null));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.quickVibration()).called(1);
|
|
verify(() => communicationBloc.add(const communication_events.MeasureEvent())).called(1);
|
|
verify(() => meteringInteractor.errorVibration()).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<LoadingState>(),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.isMetering, 'isMetering', false)
|
|
.having((state) => state.ev100, 'ev100', null)
|
|
.having((state) => state.ev, 'ev', null),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'`MeasureEvent` -> continuous metering',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
// delays here simulate light sensor behaviour
|
|
// when sensor does not fire new LUX events when value is not changed
|
|
bloc.add(const MeasureEvent());
|
|
bloc.onCommunicationState(const communication_states.MeteringInProgressState(null));
|
|
await Future.delayed(const Duration(seconds: 1));
|
|
bloc.onCommunicationState(const communication_states.MeteringInProgressState(2));
|
|
bloc.onCommunicationState(const communication_states.MeteringInProgressState(5.5));
|
|
await Future.delayed(const Duration(seconds: 2));
|
|
bloc.onCommunicationState(const communication_states.MeteringInProgressState(null));
|
|
bloc.onCommunicationState(const communication_states.MeteringInProgressState(4));
|
|
bloc.add(const MeasureEvent());
|
|
bloc.onCommunicationState(const communication_states.MeteringEndedState(4));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.quickVibration()).called(2);
|
|
verify(() => communicationBloc.add(const communication_events.MeasureEvent())).called(2);
|
|
verify(() => meteringInteractor.responseVibration()).called(4);
|
|
verify(() => meteringInteractor.errorVibration()).called(2);
|
|
},
|
|
expect: () => [
|
|
isA<LoadingState>(),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.isMetering, 'isMetering', true)
|
|
.having((state) => state.ev, 'ev', null),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.isMetering, 'isMetering', true)
|
|
.having((state) => state.ev, 'ev', 2),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.isMetering, 'isMetering', true)
|
|
.having((state) => state.ev, 'ev', 5.5),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.isMetering, 'isMetering', true)
|
|
.having((state) => state.ev, 'ev', null),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.isMetering, 'isMetering', true)
|
|
.having((state) => state.ev, 'ev', 4),
|
|
isA<LoadingState>(),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.isMetering, 'isMetering', false)
|
|
.having((state) => state.ev, 'ev', 4),
|
|
],
|
|
);
|
|
},
|
|
timeout: const Timeout(Duration(seconds: 4)),
|
|
);
|
|
|
|
group(
|
|
'`IsoChangedEvent`',
|
|
() {
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Pick different ISO (ev100 != null)',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(const IsoChangedEvent(IsoValue(200, StopType.full)));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.iso = const IsoValue(200, StopType.full)).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 1.0)
|
|
.having((state) => state.ev, 'ev', 2.0)
|
|
.having((state) => state.iso, 'iso', const IsoValue(200, StopType.full))
|
|
.having((state) => state.nd, 'nd', NdValue.values.first)
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Pick different ISO (ev100 = null)',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: null,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(const IsoChangedEvent(IsoValue(200, StopType.full)));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.iso = const IsoValue(200, StopType.full)).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', null)
|
|
.having((state) => state.ev, 'ev', null)
|
|
.having((state) => state.iso, 'iso', const IsoValue(200, StopType.full))
|
|
.having((state) => state.nd, 'nd', NdValue.values.first)
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Pick same ISO',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(const IsoChangedEvent(IsoValue(100, StopType.full)));
|
|
},
|
|
verify: (_) {
|
|
verifyNever(() => meteringInteractor.iso = const IsoValue(100, StopType.full));
|
|
},
|
|
expect: () => [],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Pick different ISO & measure',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(const IsoChangedEvent(IsoValue(200, StopType.full)));
|
|
bloc.add(const MeasureEvent());
|
|
bloc.onCommunicationState(const communication_states.MeteringEndedState(2));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.iso = const IsoValue(200, StopType.full)).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 1.0)
|
|
.having((state) => state.ev, 'ev', 2.0)
|
|
.having((state) => state.iso, 'iso', const IsoValue(200, StopType.full))
|
|
.having((state) => state.nd, 'nd', NdValue.values.first)
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
isA<LoadingState>(),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 2.0)
|
|
.having((state) => state.ev, 'ev', 3.0)
|
|
.having((state) => state.iso, 'iso', const IsoValue(200, StopType.full))
|
|
.having((state) => state.nd, 'nd', NdValue.values.first)
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`NdChangedEvent`',
|
|
() {
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Pick different ND (ev100 != null)',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(const NdChangedEvent(NdValue(2)));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.ndFilter = const NdValue(2)).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 1.0)
|
|
.having((state) => state.ev, 'ev', 0.0)
|
|
.having((state) => state.iso, 'iso', const IsoValue(100, StopType.full))
|
|
.having((state) => state.nd, 'nd', const NdValue(2))
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Pick different ND (ev100 = null)',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: null,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(const NdChangedEvent(NdValue(2)));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.ndFilter = const NdValue(2)).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', null)
|
|
.having((state) => state.ev, 'ev', null)
|
|
.having((state) => state.iso, 'iso', const IsoValue(100, StopType.full))
|
|
.having((state) => state.nd, 'nd', const NdValue(2))
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Pick same ND',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(NdChangedEvent(NdValue.values.first));
|
|
},
|
|
verify: (_) {
|
|
verifyNever(() => meteringInteractor.ndFilter = NdValue.values.first);
|
|
},
|
|
expect: () => [],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Pick different ND & measure',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(const NdChangedEvent(NdValue(2)));
|
|
bloc.add(const MeasureEvent());
|
|
bloc.onCommunicationState(const communication_states.MeteringEndedState(2));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.ndFilter = const NdValue(2)).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 1.0)
|
|
.having((state) => state.ev, 'ev', 0.0)
|
|
.having((state) => state.iso, 'iso', const IsoValue(100, StopType.full))
|
|
.having((state) => state.nd, 'nd', const NdValue(2))
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
isA<LoadingState>(),
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 2.0)
|
|
.having((state) => state.ev, 'ev', 1.0)
|
|
.having((state) => state.iso, 'iso', const IsoValue(100, StopType.full))
|
|
.having((state) => state.nd, 'nd', const NdValue(2))
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`EquipmentProfileChangedEvent`',
|
|
() {
|
|
final reducedProfile = EquipmentProfile(
|
|
id: '0',
|
|
name: 'Reduced',
|
|
apertureValues: ApertureValue.values,
|
|
ndValues: NdValue.values.getRange(0, 3).toList(),
|
|
shutterSpeedValues: ShutterSpeedValue.values,
|
|
isoValues: IsoValue.values.getRange(4, 23).toList(),
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'New profile has current ISO & ND',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(EquipmentProfileChangedEvent(reducedProfile));
|
|
},
|
|
verify: (_) {
|
|
verifyNever(() => meteringInteractor.iso = reducedProfile.isoValues.first);
|
|
verifyNever(() => meteringInteractor.ndFilter = reducedProfile.ndValues.first);
|
|
verifyNever(() => meteringInteractor.responseVibration());
|
|
},
|
|
expect: () => [],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'New profile has new ISO & current ND',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: IsoValue.values[2],
|
|
nd: NdValue.values.first,
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(EquipmentProfileChangedEvent(reducedProfile));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.iso = reducedProfile.isoValues.first).called(1);
|
|
verifyNever(() => meteringInteractor.ndFilter = reducedProfile.ndValues.first);
|
|
verify(() => meteringInteractor.responseVibration()).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 1.0)
|
|
.having((state) => state.iso, 'iso', reducedProfile.isoValues.first)
|
|
.having((state) => state.nd, 'nd', NdValue.values.first)
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'New profile has current ISO & new ND',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: const IsoValue(100, StopType.full),
|
|
nd: NdValue.values[4],
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(EquipmentProfileChangedEvent(reducedProfile));
|
|
},
|
|
verify: (_) {
|
|
verifyNever(() => meteringInteractor.iso = reducedProfile.isoValues.first);
|
|
verify(() => meteringInteractor.ndFilter = reducedProfile.ndValues.first).called(1);
|
|
verify(() => meteringInteractor.responseVibration()).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 1.0)
|
|
.having((state) => state.iso, 'iso', const IsoValue(100, StopType.full))
|
|
.having((state) => state.nd, 'nd', reducedProfile.ndValues.first)
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'New profile has new ISO & new ND',
|
|
build: () => bloc,
|
|
seed: () => MeteringDataState(
|
|
ev100: 1.0,
|
|
iso: IsoValue.values[2],
|
|
nd: NdValue.values[4],
|
|
isMetering: false,
|
|
),
|
|
act: (bloc) async {
|
|
bloc.add(EquipmentProfileChangedEvent(reducedProfile));
|
|
},
|
|
verify: (_) {
|
|
verify(() => meteringInteractor.iso = reducedProfile.isoValues.first).called(1);
|
|
verify(() => meteringInteractor.ndFilter = reducedProfile.ndValues.first).called(1);
|
|
verify(() => meteringInteractor.responseVibration()).called(1);
|
|
},
|
|
expect: () => [
|
|
isA<MeteringDataState>()
|
|
.having((state) => state.ev100, 'ev100', 1.0)
|
|
.having((state) => state.iso, 'iso', reducedProfile.isoValues.first)
|
|
.having((state) => state.nd, 'nd', reducedProfile.ndValues.first)
|
|
.having((state) => state.isMetering, 'isMetering', false),
|
|
],
|
|
);
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`Volume keys shutter action`',
|
|
() {
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Add/remove listener',
|
|
build: () => bloc,
|
|
verify: (_) {
|
|
verify(() => volumeKeysNotifier.addListener(bloc.onVolumeKey)).called(1);
|
|
verify(() => volumeKeysNotifier.removeListener(bloc.onVolumeKey)).called(1);
|
|
},
|
|
expect: () => [],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'onVolumeKey & VolumeAction.shutter',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.onVolumeKey();
|
|
},
|
|
setUp: () {
|
|
when(() => meteringInteractor.volumeAction).thenReturn(VolumeAction.shutter);
|
|
},
|
|
verify: (_) {},
|
|
expect: () => [isA<LoadingState>()],
|
|
);
|
|
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'onVolumeKey & VolumeAction.none',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.onVolumeKey();
|
|
},
|
|
setUp: () {
|
|
when(() => meteringInteractor.volumeAction).thenReturn(VolumeAction.none);
|
|
},
|
|
verify: (_) {},
|
|
expect: () => [],
|
|
);
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`SettingOpenedEvent`/`SettingsClosedEvent`',
|
|
() {
|
|
blocTest<MeteringBloc, MeteringState>(
|
|
'Settings opened & closed',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.add(const SettingsOpenedEvent());
|
|
bloc.add(const SettingsClosedEvent());
|
|
},
|
|
verify: (_) {
|
|
verify(() => communicationBloc.add(const communication_events.SettingsOpenedEvent()))
|
|
.called(1);
|
|
verify(() => communicationBloc.add(const communication_events.SettingsClosedEvent()))
|
|
.called(1);
|
|
},
|
|
expect: () => [],
|
|
);
|
|
},
|
|
);
|
|
}
|