mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-11-22 15:30:59 +00:00
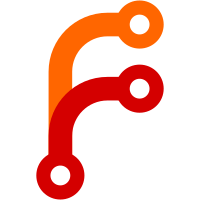
* wip * added start/stop button * animated timeline * fixed timer stop state * added reset button (wip) * added `onExposurePairTap` callback * integrated `TimerScreen` to navigation * separated `TimerTimeline` * fixed timeline flickering * added milliseconds to timer * synchronized timeline with actual timer * reused `BottomControlsBar` * fixed default scaffold background color * moved center button size to the bar itself * display selected exposure pair on timer screen * separated reusable `AnimatedCircluarButton` * release camera when timer is opened * added `TimerInteractor` * added `TimerBloc` test * fixed hours parsing * added scenarios for timer golden test * adjusted timer timeline colors * show iso & nd values on timer screen * automatically close timer screen after timeout * added timer autostart * reverted theme changes * updated goldens * typo * removed timer screen auto-dismiss * increased timer vibration duration * replaced outlined locks * increased 1/3 values font size
127 lines
4.2 KiB
Dart
127 lines
4.2 KiB
Dart
import 'package:bloc_test/bloc_test.dart';
|
|
import 'package:lightmeter/screens/metering/communication/bloc_communication_metering.dart';
|
|
import 'package:lightmeter/screens/metering/communication/event_communication_metering.dart';
|
|
import 'package:lightmeter/screens/metering/communication/state_communication_metering.dart';
|
|
import 'package:test/test.dart';
|
|
|
|
void main() {
|
|
late MeteringCommunicationBloc bloc;
|
|
|
|
setUp(() {
|
|
bloc = MeteringCommunicationBloc();
|
|
});
|
|
|
|
tearDown(() {
|
|
bloc.close();
|
|
});
|
|
|
|
group(
|
|
'`MeasureEvent`',
|
|
() {
|
|
blocTest<MeteringCommunicationBloc, MeteringCommunicationState>(
|
|
'Multiple consequtive measure events',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.add(const MeasureEvent());
|
|
bloc.add(const MeasureEvent());
|
|
bloc.add(const MeasureEvent());
|
|
bloc.add(const MeasureEvent());
|
|
},
|
|
expect: () => [
|
|
isA<MeasureState>(),
|
|
isA<MeasureState>(),
|
|
isA<MeasureState>(),
|
|
isA<MeasureState>(),
|
|
],
|
|
);
|
|
|
|
blocTest<MeteringCommunicationBloc, MeteringCommunicationState>(
|
|
'Continuous metering simulation',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.add(const MeasureEvent());
|
|
bloc.add(const MeteringInProgressEvent(1));
|
|
bloc.add(const MeteringInProgressEvent(null));
|
|
bloc.add(const MeteringInProgressEvent(null));
|
|
bloc.add(const MeteringInProgressEvent(2));
|
|
bloc.add(const MeasureEvent());
|
|
bloc.add(const MeteringEndedEvent(2));
|
|
bloc.add(const MeteringEndedEvent(2));
|
|
},
|
|
expect: () => [
|
|
isA<MeasureState>(),
|
|
isA<MeteringInProgressState>().having((state) => state.ev100, 'ev100', 1),
|
|
isA<MeteringInProgressState>().having((state) => state.ev100, 'ev100', null),
|
|
isA<MeteringInProgressState>().having((state) => state.ev100, 'ev100', 2),
|
|
isA<MeasureState>(),
|
|
isA<MeteringEndedState>().having((state) => state.ev100, 'ev100', 2),
|
|
],
|
|
);
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`MeteringInProgressEvent`',
|
|
() {
|
|
blocTest<MeteringCommunicationBloc, MeteringCommunicationState>(
|
|
'Multiple consequtive in progress events',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.add(const MeteringInProgressEvent(1));
|
|
bloc.add(const MeteringInProgressEvent(1));
|
|
bloc.add(const MeteringInProgressEvent(1));
|
|
bloc.add(const MeteringInProgressEvent(null));
|
|
bloc.add(const MeteringInProgressEvent(null));
|
|
bloc.add(const MeteringInProgressEvent(2));
|
|
},
|
|
expect: () => [
|
|
isA<MeteringInProgressState>().having((state) => state.ev100, 'ev100', 1),
|
|
isA<MeteringInProgressState>().having((state) => state.ev100, 'ev100', null),
|
|
isA<MeteringInProgressState>().having((state) => state.ev100, 'ev100', 2),
|
|
],
|
|
);
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`MeteringEndedEvent`',
|
|
() {
|
|
blocTest<MeteringCommunicationBloc, MeteringCommunicationState>(
|
|
'Multiple consequtive ended events',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.add(const MeteringEndedEvent(1));
|
|
},
|
|
expect: () => [
|
|
isA<MeteringEndedState>().having((state) => state.ev100, 'ev100', 1),
|
|
],
|
|
);
|
|
},
|
|
);
|
|
|
|
group(
|
|
'`ScreenOnTopOpenedEvent`/`ScreenOnTopClosedEvent`',
|
|
() {
|
|
blocTest<MeteringCommunicationBloc, MeteringCommunicationState>(
|
|
'Multiple consequtive settings events',
|
|
build: () => bloc,
|
|
act: (bloc) async {
|
|
bloc.add(const ScreenOnTopOpenedEvent());
|
|
bloc.add(const ScreenOnTopOpenedEvent());
|
|
bloc.add(const ScreenOnTopOpenedEvent());
|
|
bloc.add(const ScreenOnTopClosedEvent());
|
|
bloc.add(const ScreenOnTopClosedEvent());
|
|
bloc.add(const ScreenOnTopClosedEvent());
|
|
bloc.add(const ScreenOnTopOpenedEvent());
|
|
bloc.add(const ScreenOnTopClosedEvent());
|
|
},
|
|
expect: () => [
|
|
isA<SettingsOpenedState>(),
|
|
isA<SettingsClosedState>(),
|
|
isA<SettingsOpenedState>(),
|
|
isA<SettingsClosedState>(),
|
|
],
|
|
);
|
|
},
|
|
);
|
|
}
|