mirror of
https://github.com/vodemn/m3_lightmeter.git
synced 2024-10-18 14:20:40 +00:00
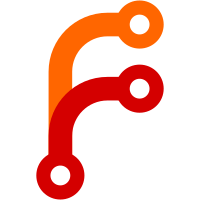
* wip * added start/stop button * animated timeline * fixed timer stop state * added reset button (wip) * added `onExposurePairTap` callback * integrated `TimerScreen` to navigation * separated `TimerTimeline` * fixed timeline flickering * added milliseconds to timer * synchronized timeline with actual timer * reused `BottomControlsBar` * fixed default scaffold background color * moved center button size to the bar itself * display selected exposure pair on timer screen * separated reusable `AnimatedCircluarButton` * release camera when timer is opened * added `TimerInteractor` * added `TimerBloc` test * fixed hours parsing * added scenarios for timer golden test * adjusted timer timeline colors * show iso & nd values on timer screen * automatically close timer screen after timeout * added timer autostart * reverted theme changes * updated goldens * typo * removed timer screen auto-dismiss * increased timer vibration duration * replaced outlined locks * increased 1/3 values font size
67 lines
1.9 KiB
Dart
67 lines
1.9 KiB
Dart
import 'package:flutter/material.dart';
|
|
import 'package:flutter_bloc/flutter_bloc.dart';
|
|
import 'package:lightmeter/data/models/exposure_pair.dart';
|
|
import 'package:lightmeter/interactors/timer_interactor.dart';
|
|
import 'package:lightmeter/providers/services_provider.dart';
|
|
import 'package:lightmeter/screens/timer/bloc_timer.dart';
|
|
import 'package:lightmeter/screens/timer/screen_timer.dart';
|
|
import 'package:m3_lightmeter_resources/m3_lightmeter_resources.dart';
|
|
|
|
class TimerFlowArgs {
|
|
final ExposurePair exposurePair;
|
|
final IsoValue isoValue;
|
|
final NdValue ndValue;
|
|
|
|
const TimerFlowArgs({
|
|
required this.exposurePair,
|
|
required this.isoValue,
|
|
required this.ndValue,
|
|
});
|
|
}
|
|
|
|
class TimerFlow extends StatelessWidget {
|
|
final TimerFlowArgs args;
|
|
late final _duration =
|
|
Duration(milliseconds: (args.exposurePair.shutterSpeed.value * Duration.millisecondsPerSecond).toInt());
|
|
|
|
TimerFlow({required this.args, super.key});
|
|
|
|
@override
|
|
Widget build(BuildContext context) {
|
|
return TimerInteractorProvider(
|
|
data: TimerInteractor(
|
|
ServicesProvider.of(context).userPreferencesService,
|
|
ServicesProvider.of(context).hapticsService,
|
|
),
|
|
child: BlocProvider(
|
|
create: (context) => TimerBloc(
|
|
TimerInteractorProvider.of(context),
|
|
_duration,
|
|
),
|
|
child: TimerScreen(
|
|
exposurePair: args.exposurePair,
|
|
isoValue: args.isoValue,
|
|
ndValue: args.ndValue,
|
|
duration: _duration,
|
|
),
|
|
),
|
|
);
|
|
}
|
|
}
|
|
|
|
class TimerInteractorProvider extends InheritedWidget {
|
|
final TimerInteractor data;
|
|
|
|
const TimerInteractorProvider({
|
|
required this.data,
|
|
required super.child,
|
|
super.key,
|
|
});
|
|
|
|
static TimerInteractor of(BuildContext context) {
|
|
return context.findAncestorWidgetOfExactType<TimerInteractorProvider>()!.data;
|
|
}
|
|
|
|
@override
|
|
bool updateShouldNotify(TimerInteractorProvider oldWidget) => false;
|
|
}
|